Some times we have to implement custom marker instead of google default location marker.So we need some extra work for that implementation. Here is the way to design custom marker in google map.
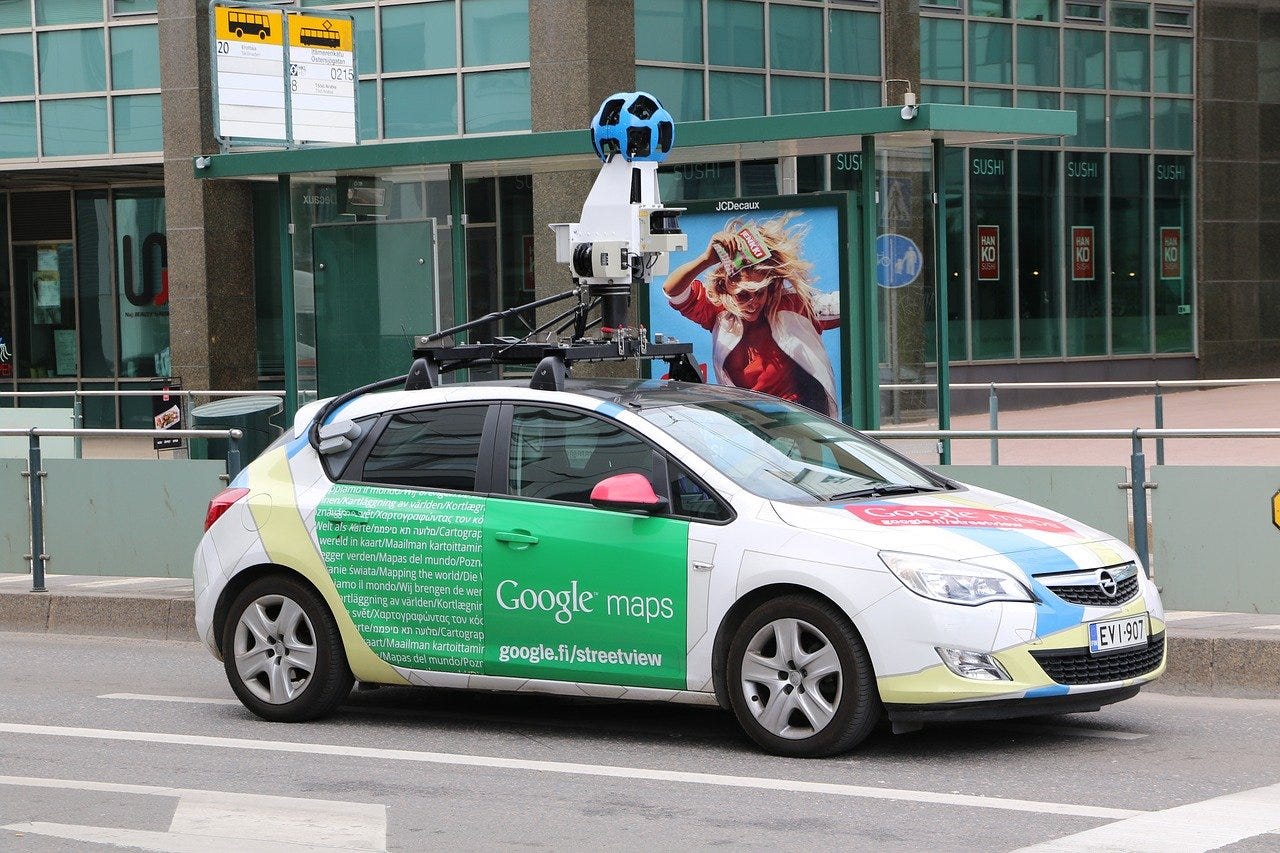
Custom marker custom_marker.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.appcompat.widget.LinearLayoutCompat xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="custom_marker"
android:layout_margin="6dp"/>
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:src="@mipmap/ic_launcher" />
</androidx.appcompat.widget.LinearLayoutCompat>
Main activity layout activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<fragment xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
android:id="@+id/map"
tools:context=".MapsActivity"
android:name="com.google.android.gms.maps.SupportMapFragment" />
</androidx.constraintlayout.widget.ConstraintLayout>
Main Activity code
import android.graphics.Bitmap
import android.graphics.Canvas
import android.graphics.Color
import android.graphics.PorterDuff
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.view.View
import com.google.android.gms.maps.CameraUpdateFactory
import com.google.android.gms.maps.GoogleMap
import com.google.android.gms.maps.OnMapReadyCallback
import com.google.android.gms.maps.SupportMapFragment
import com.google.android.gms.maps.model.BitmapDescriptorFactory
import com.google.android.gms.maps.model.CameraPosition
import com.google.android.gms.maps.model.LatLng
import com.google.android.gms.maps.model.MarkerOptions
class MainActivity : AppCompatActivity(), OnMapReadyCallback {
private lateinit var map: GoogleMap
private val sydney = LatLng(-34.0, 151.0)
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// Obtain the SupportMapFragment and get notified when the map is ready to be used.
val mapFragment = supportFragmentManager
.findFragmentById(R.id.map) as SupportMapFragment
mapFragment.getMapAsync(this)
}
override fun onMapReady(googleMap: GoogleMap) {
map = googleMap
// Add a marker in Sydney and move the camera
applyMapCamera()
val markerOptions = MarkerOptions().position(sydney).icon(
BitmapDescriptorFactory.fromBitmap(
loadBitmapView()
)
).zIndex(10f).anchor(0.5f, 0.5f)
googleMap.addMarker(markerOptions)
}
private fun applyMapCamera() {
val cameraPosition = CameraPosition.Builder()
.target(sydney) // Sets the center of the map
.zoom(13.0f) // Sets the zoom
.build()
map.animateCamera(
CameraUpdateFactory.newCameraPosition(cameraPosition)
)
}
private fun loadBitmapView(): Bitmap {
val customMarkerView = layoutInflater.inflate(R.layout.custom_marker, null)
customMarkerView.measure(View.MeasureSpec.UNSPECIFIED, View.MeasureSpec.UNSPECIFIED)
customMarkerView.layout(
0, 0,
customMarkerView.measuredWidth,
customMarkerView.measuredHeight
)
val bitmap = Bitmap.createBitmap(
customMarkerView.measuredWidth, customMarkerView.measuredHeight,
Bitmap.Config.ARGB_8888
)
val canvas = Canvas(bitmap)
canvas.drawColor(Color.WHITE, PorterDuff.Mode.SRC_IN)
customMarkerView.background?.draw(canvas)
customMarkerView.draw(canvas)
return bitmap
}
}